What3words is an interesting alternative to sharing around long latitude/longitude numbers, instead share just a few words!
The first step to integrating the api is signing up for a free API key. Then require the library using composer – in the main project folder run:
composer require what3words/w3w-php-wrapper
The library should install. You may need to install mbstring or other libraries if you get an error – for example:
sudo apt install php*mbstring
Then run the composer require command again as shown above.
Now with this installed, we can add a call to look up coordinates by the three word address, as explained in their php documentation. First a route should be created in routes/api.php for an external /api url to call:
Route::get('/mylookup/v1', 'What3WordsController@lookup');
A controller class will output the result, and can be started with:
php artisan make:controller What3WordsController
Now you will see a file in Http/Controllers folder, which you can add the “lookup” function referenced above. Add the lookup function:
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use What3words\Geocoder\Geocoder; /// ADDED for this file to reference What3words
class What3WordsController extends Controller
{
function lookup(Request $request)
{
$api = new Geocoder("YOURAPIKEY");
$result = $api->convertToCoordinates($request->words);
return response()->json($result, 200);
}
}
Using API in Ajax or Apps
Now run “php artisan serve” if you don’t have this up and running in your browser – Go to “http://127.0.0.1:8000/api/mylookup/v1/?words=filled.count.soap” to look up the example location in the api, you will get a json object back:
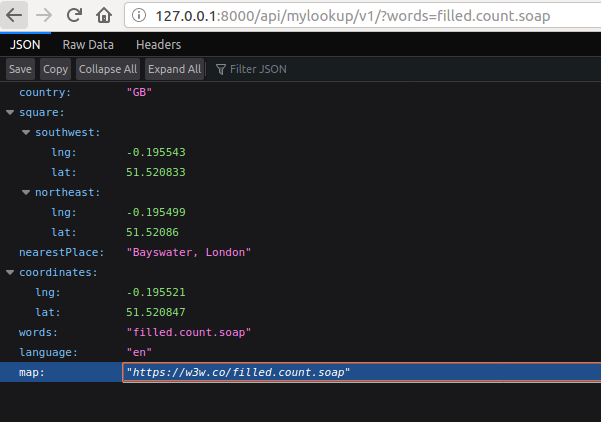
Now if you need this in some AJAX application you can look this up within your web app. For example, after including jQuery, you can look up using:
jQuery.get('/api/mylookup/v1/',{words:'filled.count.soap'}, function(info){console.log(info.coordinates.lat); console.log(info.coordinates.lng);}, 'JSON')
for example.
I’ve integrated this feature and the HearHam Live Amateur Radio Repeater Listing now allows searching for nearby repeaters by what3words address – check it out!
Update: The new update of the desktop app Repeater-START now lets you search and go directly to a What3words location in the search bar!
You should put this in the register method of your AppServiceProvider.php
$this->app->singleton(Geocoder::class, function() {
return new Geocoder(env(‘W3W_API_KEY’));
});
And that way you can use dependency injection instead of constructing a new object every time you need it in a controller:
public function __construct(
public Geocoder $w3w
){}
I had not tried that before. Looks interesting: https://laravel.com/docs/7.x/container#binding